TL;DR:
🔄 Redux provides a predictable, centralized way to manage state in large-scale ReactJS applications.
🧠 Core Redux concepts include Store, Actions, Reducers, and Dispatch—key to understanding the unidirectional data flow.
⚙️ Redux outperforms Context API in handling complex state logic, middleware, and large app performance.
🛠️ With redux-thunk, setting up async data flows like API calls becomes straightforward.
👨💻 Strong TypeScript support and Redux DevTools enhance maintainability and debugging efficiency.
Introduction
React’s component-based architecture is powerful and flexible. But as applications scale, managing and sharing state across components becomes complex. That’s where Redux steps in, offering a predictable way to manage state globally.
What is ReactJS with Redux?
Redux is a predictable state container designed specifically for JavaScript applications, particularly beneficial when working with ReactJS. As a ReactJS Development Services Company, implementing Redux in React applications provides a centralized approach to state management. The combination of ReactJS with Redux creates a powerful architecture where the application’s state is stored in a single source of truth called the store. This setup enables better control over data flow and makes state changes more predictable and traceable.
Core Concepts of Redux:
– Store: Central place that holds the app’s state.
– Actions: Plain JS objects that describe what happened.
– Reducers: Pure functions that determine how the state changes based on actions.
– Dispatch: Sends actions to the reducer to update the state.
Redux Flow Diagram
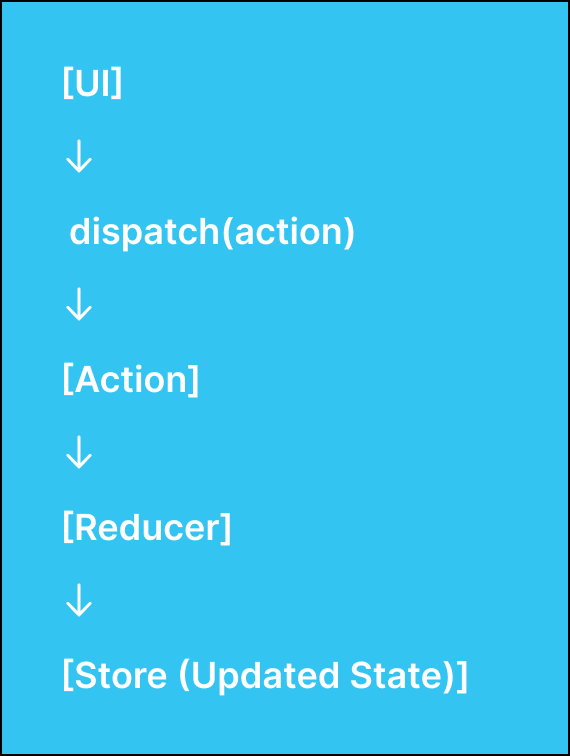
Read More: Top 9 React Component Libraries
Benefits of Using Redux for State Management in React
- Centralized State Management: All application data is stored in a single store, making it easier to track and manage state changes.
- Predictable State Updates: Redux follows a strict unidirectional data flow, ensuring consistent state updates across the application.
- Enhanced Debugging: Redux DevTools provide powerful debugging capabilities, including time-travel debugging.
- Improved Performance: Redux’s connect() function optimizes component rendering, preventing unnecessary re-renders.
Comparing Redux with Context API
While both Redux and Context API serve state management purposes, they have distinct characteristics:
- Performance: Redux offers better performance optimization through selective component updates.
- Middleware Support: Redux provides robust middleware support for handling side effects and async operations.
- Learning Curve: Context API has a gentler learning curve but lacks Redux’s advanced features.
- Scale: Redux is better suited for large-scale applications with complex state management needs.
Redux Tutorial: Integrating Redux into Your ReactJS Application
Setting Up the Redux Store in React
To begin implementing ReactJS with Redux, follow these steps:
- Use the command below to install Redux + Thunk:
npm install redux react-redux redux-thunk axios
- Redux Setup
// store.ts
import { createStore, applyMiddleware, combineReducers } from 'redux';
import thunk from 'redux-thunk';
import { userReducer } from './userReducer';
const rootReducer = combineReducers({
users: userReducer,
});
const store = createStore(rootReducer, applyMiddleware(thunk));
export default store;
export type RootState = ReturnType<typeof rootReducer>;
//userActions.ts
import axios from 'axios';
import { Dispatch } from 'redux';
export const FETCH_USERS_REQUEST = 'FETCH_USERS_REQUEST';
export const FETCH_USERS_SUCCESS = 'FETCH_USERS_SUCCESS';
export const FETCH_USERS_FAILURE = 'FETCH_USERS_FAILURE';
export const fetchUsers = () => {
return async (dispatch: Dispatch) => {
dispatch({ type: FETCH_USERS_REQUEST });
try {
const response = await axios.get('https://jsonplaceholder.typicode.com/users');
dispatch({ type: FETCH_USERS_SUCCESS, payload: response.data });
} catch (error) {
dispatch({ type: FETCH_USERS_FAILURE, error: error.message });
}
};
};
// userReducer.ts
import {
FETCH_USERS_REQUEST,
FETCH_USERS_SUCCESS,
FETCH_USERS_FAILURE,
} from './userActions';
const initialState = {
loading: false,
users: [],
error: '',
};
export const userReducer = (state = initialState, action: any) => {
switch (action.type) {
case FETCH_USERS_REQUEST:
return { ...state, loading: true };
case FETCH_USERS_SUCCESS:
return { loading: false, users: action.payload, error: '' };
case FETCH_USERS_FAILURE:
return { loading: false, users: [], error: action.error };
default:
return state;
}
};
- Provide Store in App
//index.tsx
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import App from './App';
import store from './store';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
- Create UserList Component
//index.tsx
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import App from './App';
import store from './store';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Conclusion
Mastering ReactJS with Redux is essential for building scalable and maintainable applications. By following this comprehensive guide, you’ve learned how to implement Redux in React applications effectively. Whether you’re working with a web development company or planning to hire ReactJS developers, understanding these concepts will help you create robust applications with efficient state management.
FAQs
- Why should I use Redux with React?
Redux provides predictable state management and is ideal for large applications with complex state requirements. - Is Redux only for React?
No. Redux is a standalone library and can be used with any JS framework like Angular or Vue. - What is the best middleware for Redux async?
Redux-thunk is beginner-friendly; Redux-saga is more powerful for complex async workflows. - Should I use Redux or Context API?
Choose Redux for large applications with complex state management needs, and Context API for simpler applications. - What are the core principles of Redux?
Redux follows three main principles:
- Single Source of Truth – The entire state is stored in one object.
- State is Read-Only – The only way to change the state is by dispatching actions.
- Changes are made with Pure Functions – Reducers are pure functions that return a new state.
- Is Redux still relevant with modern React (Hooks, Context, etc.)?
Yes. While Hooks and Context simplify local state, Redux is still preferred for managing complex, global state with tools like Redux Toolkit. - What is Redux Toolkit, and should I use it?
Redux Toolkit is the official, recommended way to write Redux logic. It reduces boilerplate and adds helpful utilities for slices, async thunks, and more. - How does Redux improve debugging and development workflow?
Redux integrates seamlessly with Redux DevTools, allowing you to track actions, time-travel debug, and visualize state changes. - Can I use Redux with TypeScript?
Absolutely. Redux and Redux Toolkit have strong TypeScript support, helping create strongly typed actions, reducers, and selectors for better maintainability.